Creating The Game Spec¶
- First, go to the “My Games” tab on the home page and click on “Create New” to create a new game.
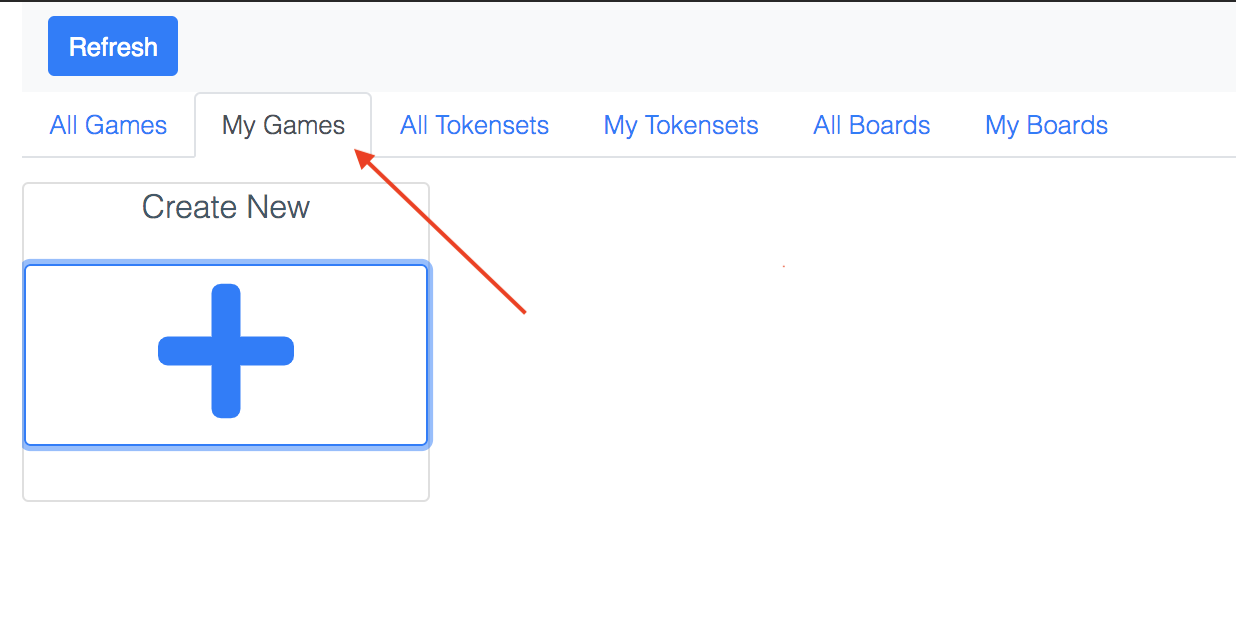
- You should now see your game in the list of games.
- Click on “Details” on your game card to open the game spec editor.
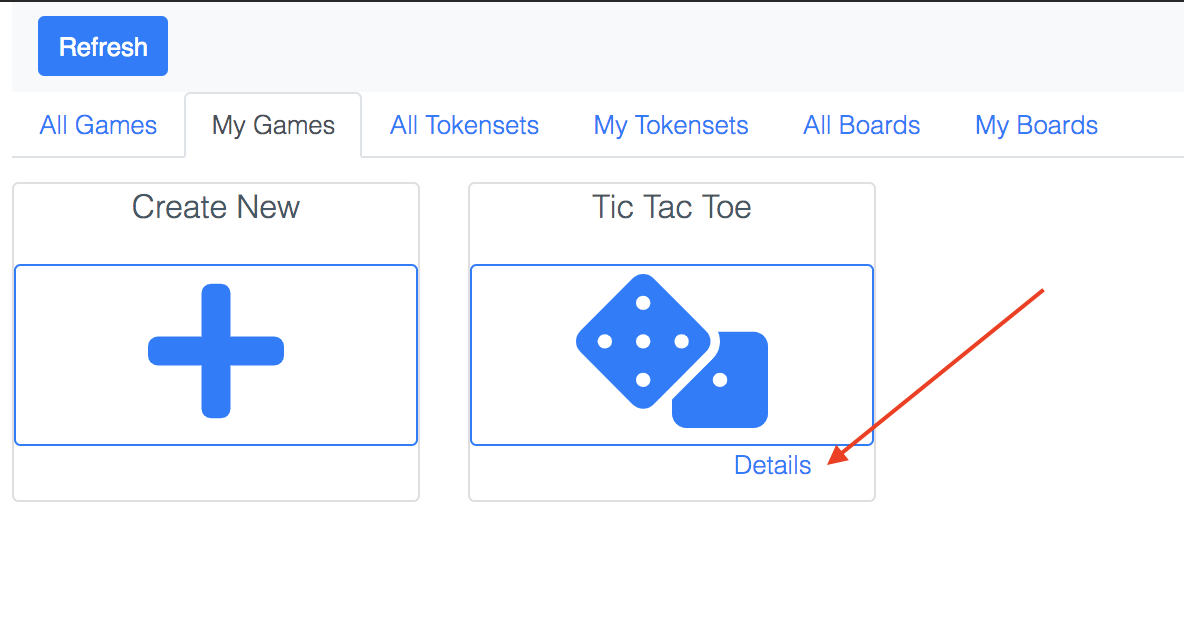
- Set “Num players” to 2
- For now, ignore Tokenset, Board, and Rules, we’ll fill them in later.
- If you like, pick a fontawesome icon to use as the game icon
Setting Up Initial Gamestate¶
For details on how Turn Base keeps track of game state, see here.
During play, the Turnbase backend keeps an internal representation of the game state. The game state is a hierarchical structure consisting of PROPs, CONTAINERs, and TOKENs <token>`
The “Initial state JSON” specifies the game state at the start of the game. At this point, the game designer should think about how the game state is going to be represented.
On paper, tic-tac-toe is played by drawing x’s and o’s into a grid. We can make this into a board game by pretending that the players place “x” and “o” tokens into a grid instead. Each space on the grid is a CONTAINER.
We need the following:
- 9 containers
- We will want to know the coordinates of the containers to facilitate computing winning logic. This can be specified in the container props.
- containers to keep each player’s tokens (they need to come from somewhere)
- The game engine expects the game state to have a “play_order” prop representing the relative order between players
Play Order¶
Let’s set up the play order first. Because the play order is required, this is the minimal possible legal game state:
{
"containers": {}
"props": {
"play_order": []
}
}
(You can omit containers and the BE will fill it in with an empty JSON, but it’s good practice to be explicit.)
For tic-tac-toe, we can just call the players “x” and “o”, after the type of token they will be placing. So the game state should look like this
{
"containers": {}
"props": {
"play_order": ["x", "o"]
}
}
If you need to, you can randomize the starting player later.
Note
The number of items in play_order
should match the Num players
setting
Adding Containers¶
As we said, we’ll need 9 containers arranged in a grid. So we’ll put in something like this
{
"containers": {
"00": {
"props": {
"i": 0,
"j": 0,
"visible_to": [
"x",
"o",
"observer"
],
"name": "00",
"type": "container"
},
"contents": []
},
"01": {
"props": {
"i": 0,
"j": 1,
"visible_to": [
"x",
"o",
"observer"
],
"name": "01",
"type": "container"
},
"contents": []
},
...
"22": {
"props": {
"i": 2,
"j": 2,
"visible_to": [
"x",
"o",
"observer"
],
"name": "22",
"type": "container"
},
"contents": []
},
"x_tokens": {
"props": {
"name": "x_tokens",
"type": "container"
},
"contents": []
},
"o_tokens": {
"props": {
"name": "o_tokens",
"type": "container"
},
"contents": []
}
},
"props": {
"play_order": [
"x",
"o"
]
}
}
- A CONTAINER has a set of PROPS as well as a list of TOKENs indexed under
contents
.- In the beginning of the game, we’ll leave all the containers empty, so
contents
is set to[]
.
- In the beginning of the game, we’ll leave all the containers empty, so
- Under
props
, we can specify any relevant information for that container. Here we want to specify its coordinates. visible_to
is a special PROP that determines which players (if any) can see the contents of that container.- For example, in a game like Spades, a player’s hand should only be visible to that player. As tic-tac-toe is perfect information, all container should be visible to eveyrone.
- “observer” refers to anyone in the room who is not a player
- We set up special containers
x_tokens
ando_tokens
to keep all of the tokens that the players will be using to play the game.