Spades Tutorial Part 1¶
Initial State JSON¶
See initial state json for a more detailed explanation of what this is, but for now there are just a few things to note:
play_order
is['player_0', 'player_1', 'player_2', 'player_3']
, so these are the names of the players in this game- There are containers for each of the players for their hand and their immediate play, like
player_0_hand
andplayer_0_play
- There is a container
draw_deck
that contains all the cards, in order. - There are a few props that hold useful information, like
suit_led
,current_trick_count
, and so on.
Setting Up the Game¶
When the game starts, a special function called set_up
is called. Let’s go ahead and define that function now.
For Spades, what we want to do is shuffle the deck of cards and deal 13 to each player. So, that code looks like this:
1 2 3 4 5 6 7 8 9 10 11 12 13 | DEF set_up:
SHUFFLE GET_CONTAINER 'draw_deck';
ASSIGN 'i' 3;
WHILE GREATER_OR_EQUAL $i 0:
ASSIGN 'j' 12;
WHILE GREATER_OR_EQUAL $j 0:
ASSIGN 'tmp' POP GET_CONTAINER 'draw_deck' 0;
APPEND GET_CONTAINER CONCAT CONCAT 'player_' STR $i '_hand' $tmp;
ASSIGN 'j' PLUS $j -1;
END_WHILE
ASSIGN 'i' PLUS $i -1;
END_WHILE
END_DEF
|
Line 2 shuffles the contents of the container draw_deck
, which contained our initial deck of cards. The rest of the code loops over two variables i and j to deal out 13 cards from draw_deck
to a player’s hand.
For more details on what’s happening here, check out the LudL Language Manual.
Test Set Up¶
Go ahead and paste the above code into the ruleset (keeping the receiver we had from before). Now the game is able to set up (though it can’t do anything else yet), which you can verify by creating a new game room from its game lobby:
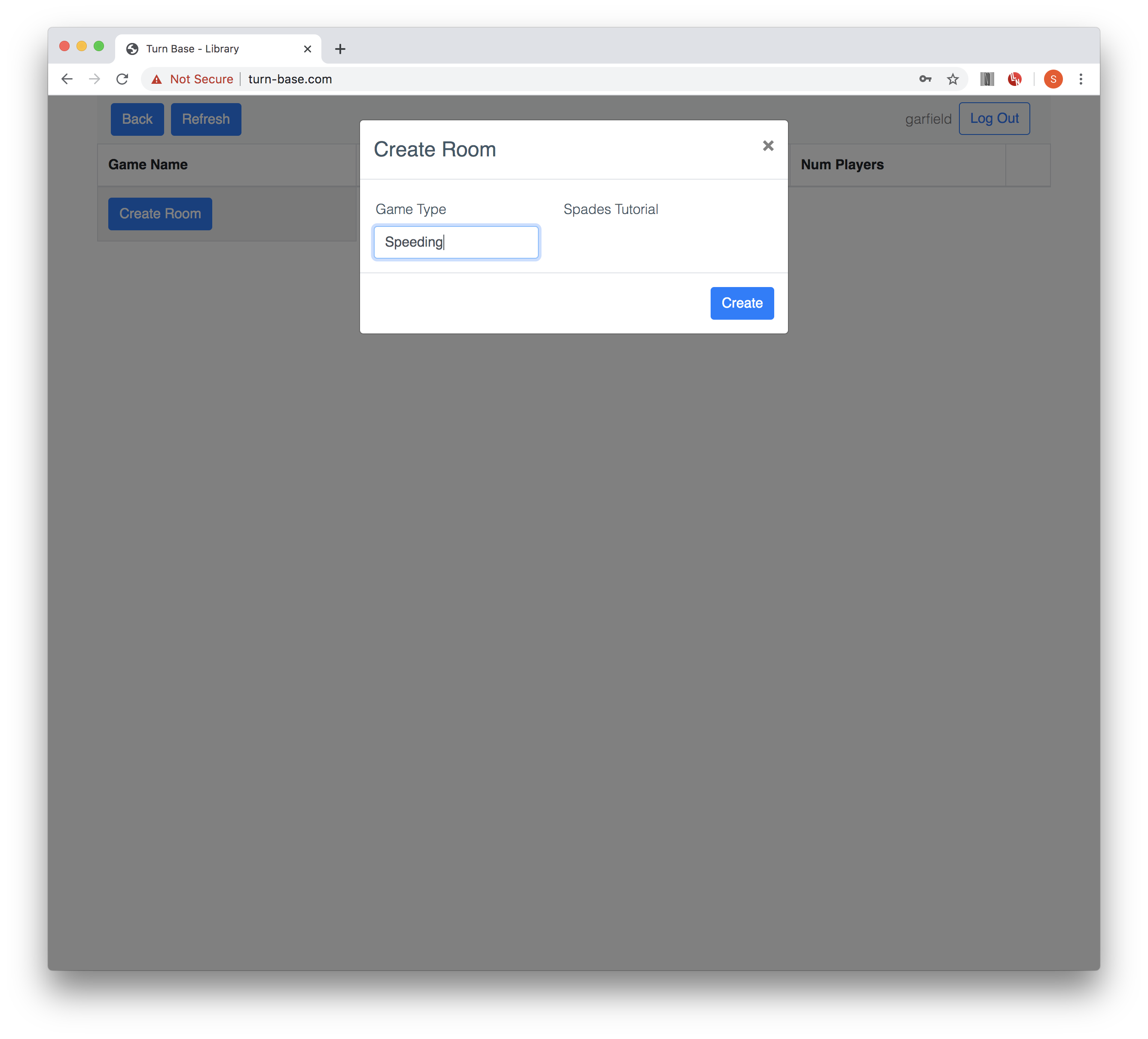
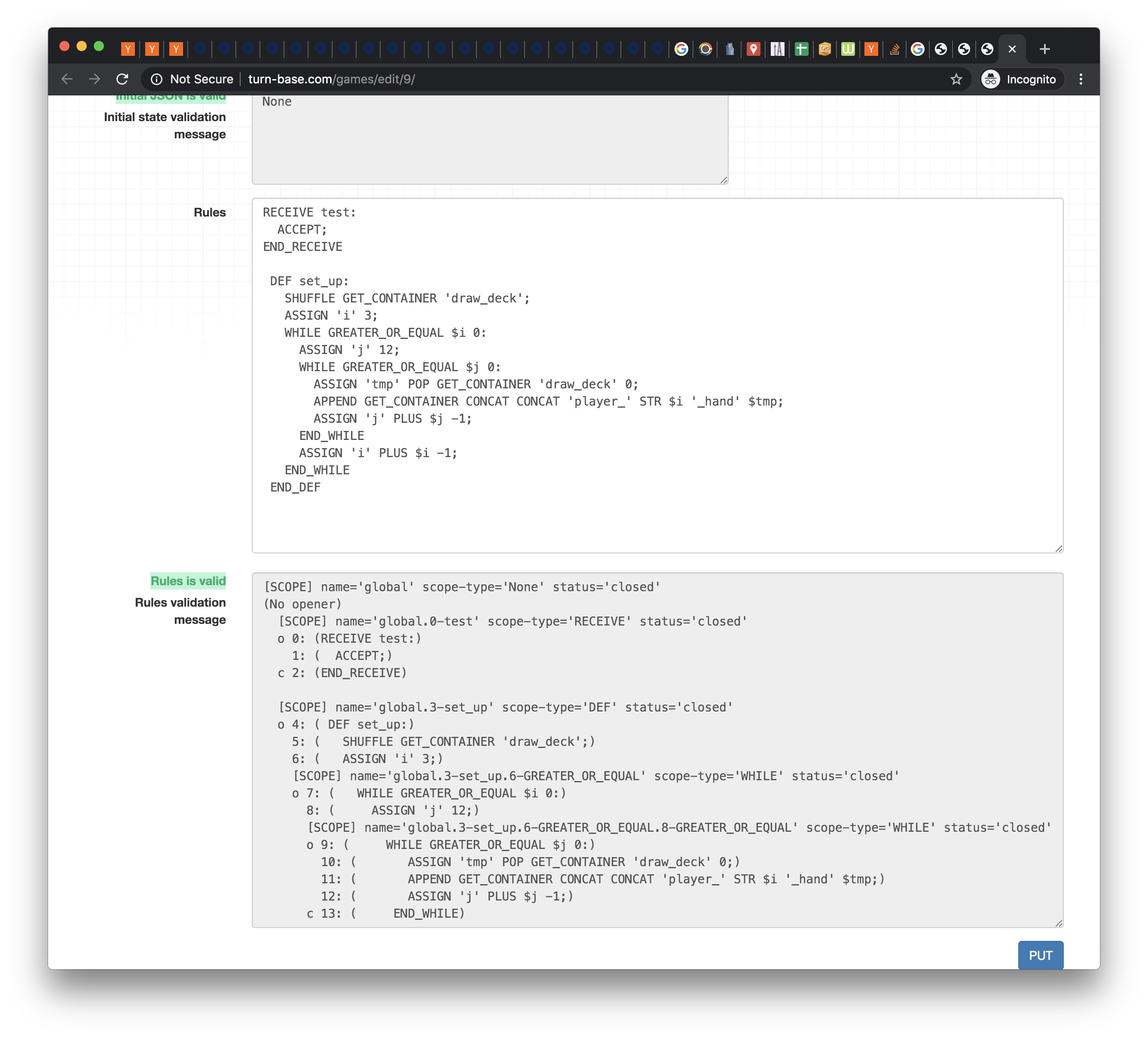
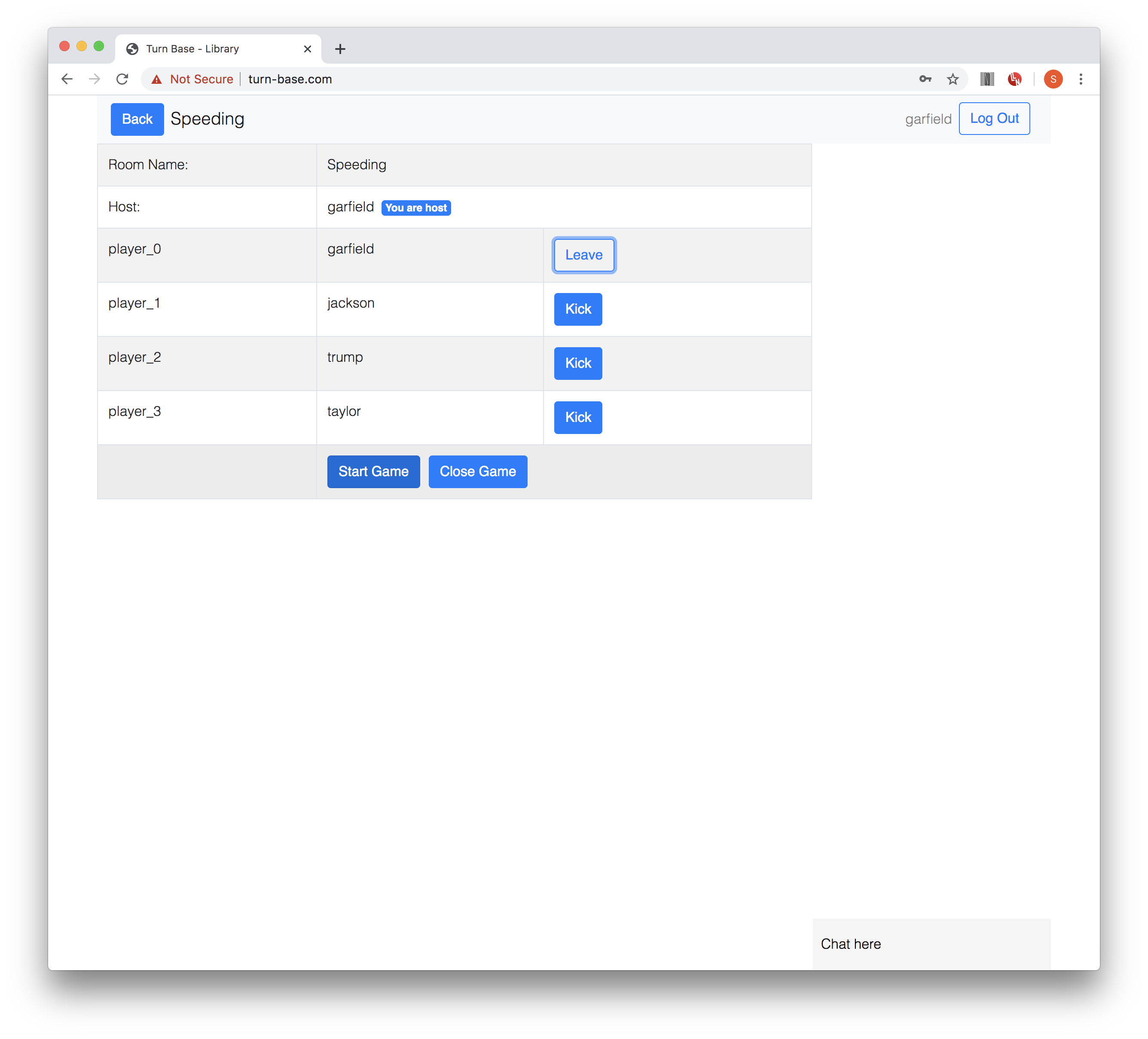
Clicking on “Start Game” loads the initial state JSON and then executes set_up
:
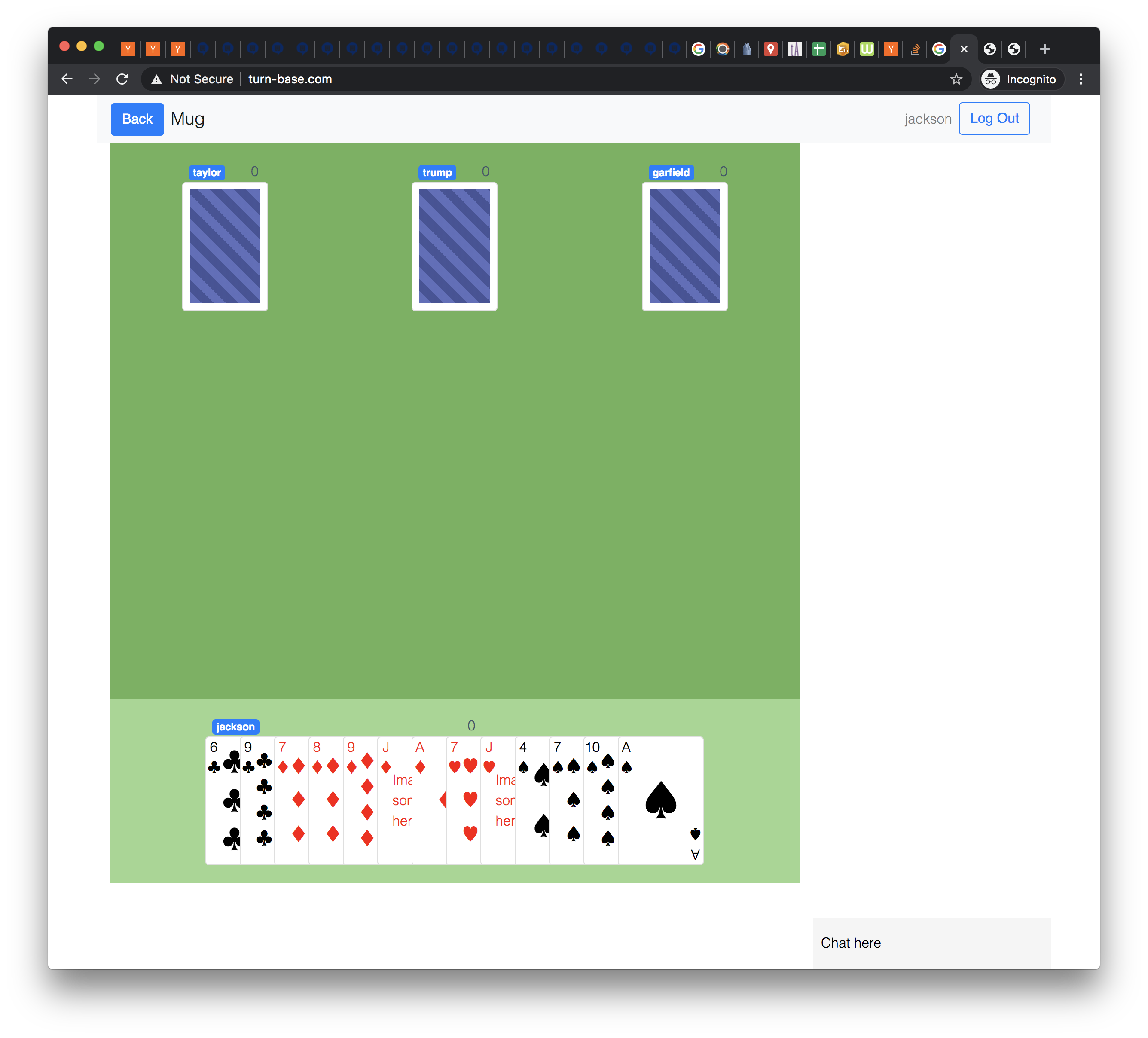
However, we haven’t implemented any game logic, so trying to play cards results in an error:
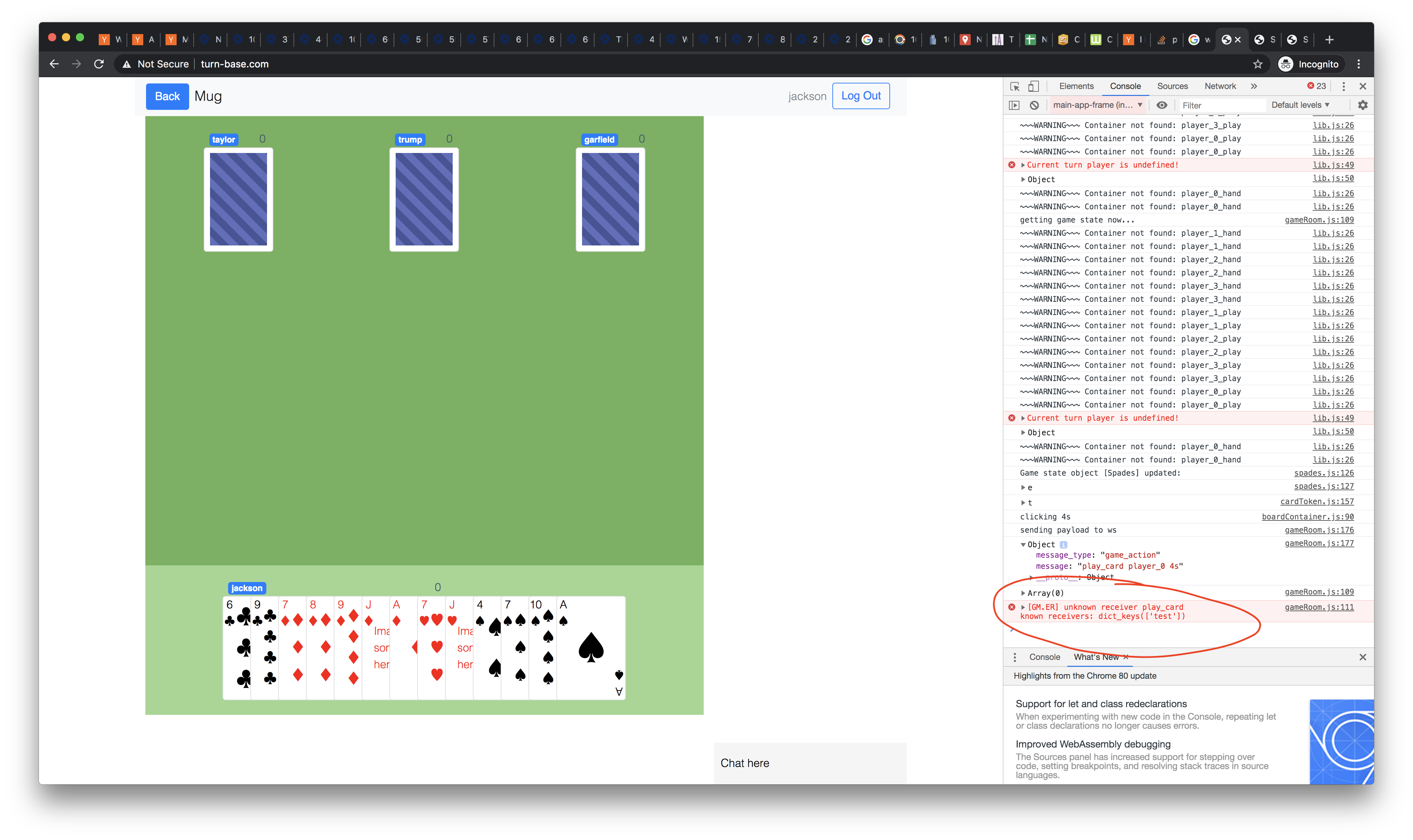